Android Image Annotation SDK
System Compatibility#
The Annotation Editor SDK for Android requires Android 5+ (SDK 21) or later. The SDK is optimized for modern Android versions, ensuring compatibility and high performance across a wide range of devices.
Key Capabilities of the Android Annotation Editor SDK#
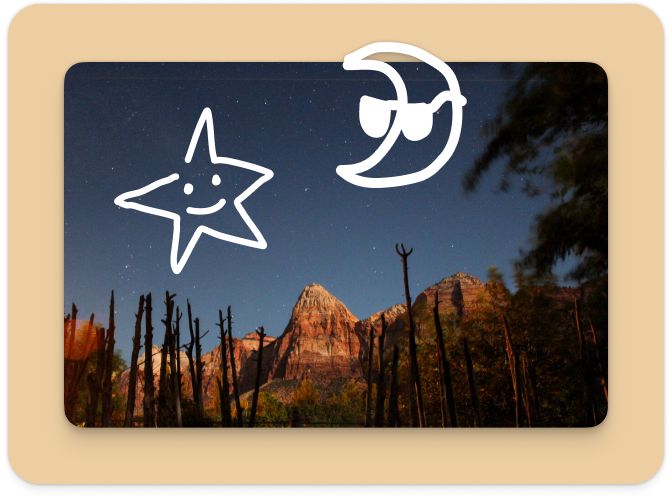
Brush Tool
Enable flexible marking and colored highlights on photos.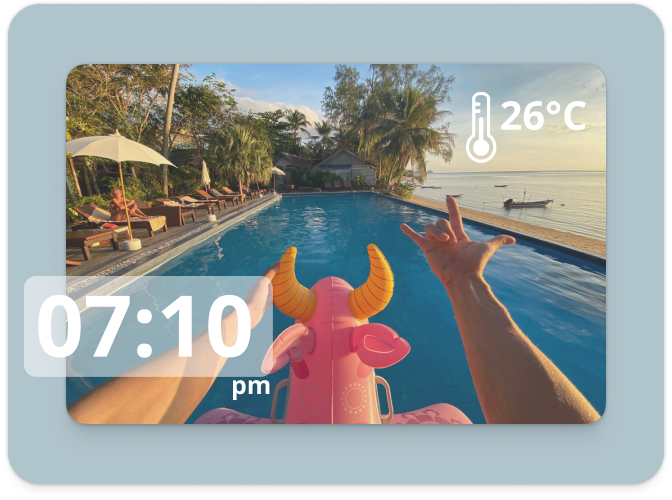
Sticker Tool
Add shapes such as arrows, tags, or badges for clear and effective annotations.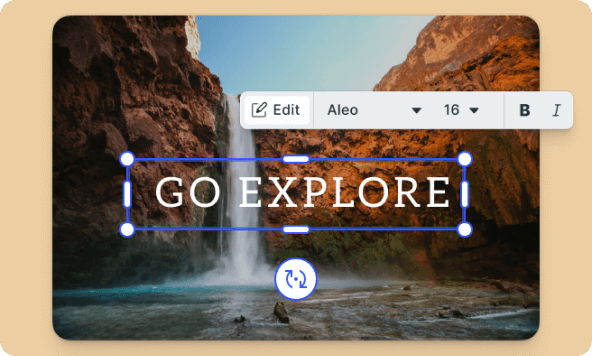
Text Tool
Provide additional information around annotation markers with custom text.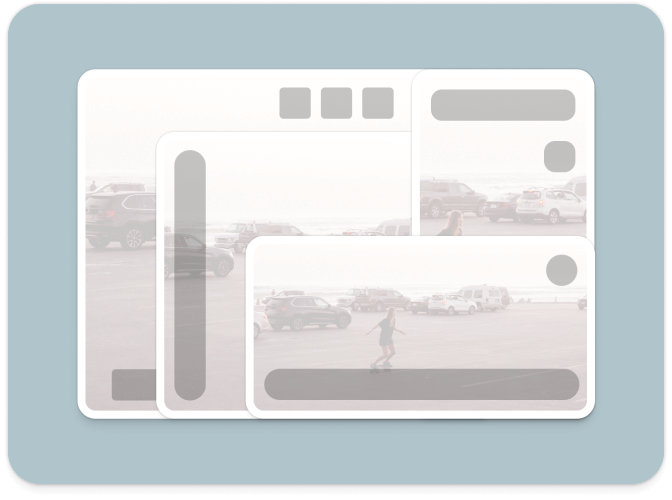
Customizable Sticker Sets
Configure the editor to include only annotation-specific stickers, ensuring a focused and streamlined user experience.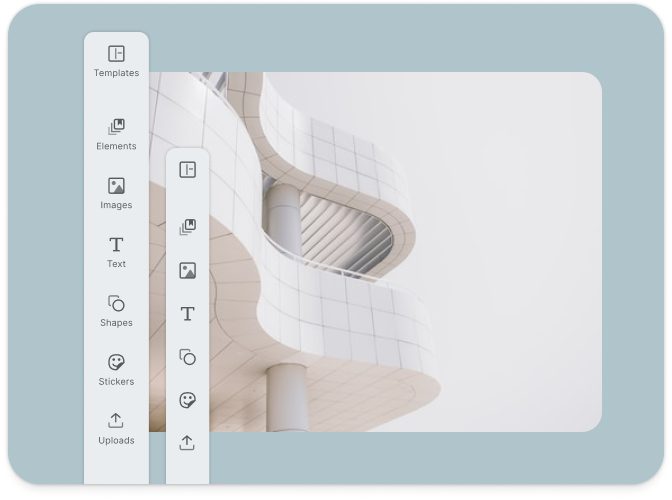
Configurable Menu Items
Tailor the editor’s menu to display only the tools necessary for annotation, simplifying the user interface.Essential Guides#
To help you get started and fully leverage the Android Annotation Editor SDK, we've compiled a set of essential guides:
- How to Get Started: Integrate the editor into your project and configure it for annotation tasks.
- How to Configure the Menu Items: Learn how to include only the annotation-specific tools in the editor’s menu using the
ToolItem
API. - How to Customize Stickers: Set up custom sticker categories with shapes such as arrows and badges for effective annotations.
- How to Use the Brush Tool: Implement the brush tool to allow users to highlight and mark areas of interest on photos.
- Understanding the SDK’s Architecture: Gain insights into the underlying architecture of the SDK to better understand its capabilities and how to utilize them.
- How to Handle Export and Errors: Manage the export process and handle errors gracefully within the annotation editor.
Customizing the Android Annotation Editor SDK#
You can tailor the Annotation Editor SDK to fit your app’s specific annotation needs in several ways:
- Configuring the Tool Menu: Use the
ToolItem
API to include only the brush, sticker, and text tools, creating a focused annotation experience. - Custom Sticker Categories: Create
ImageStickerItems
from local drawable resources, such as arrows and badges, and group them meaningfully in the editor UI. - Asset Configuration: Configure the
PhotoEditorSettingsList
to include only the stickers relevant to annotations, ensuring that the editor remains simple and user-friendly.
Example Code#
Here's an example of how to configure the Annotation Editor SDK for Android:
package ly.img.catalog.examples.solutions.pesdkimport android.content.Intentimport androidx.appcompat.app.AppCompatActivityimport ly.img.android.pesdk.PhotoEditorSettingsListimport ly.img.android.pesdk.backend.decoder.ImageSourceimport ly.img.android.pesdk.backend.model.EditorSDKResultimport ly.img.android.pesdk.backend.model.state.LoadSettingsimport ly.img.android.pesdk.ui.activity.PhotoEditorBuilderimport ly.img.android.pesdk.ui.model.state.UiConfigMainMenuimport ly.img.android.pesdk.ui.model.state.UiConfigStickerimport ly.img.android.pesdk.ui.panels.BrushToolPanelimport ly.img.android.pesdk.ui.panels.StickerToolPanelimport ly.img.android.pesdk.ui.panels.TextToolPanelimport ly.img.android.pesdk.ui.panels.item.ImageStickerItemimport ly.img.android.pesdk.ui.panels.item.StickerCategoryItemimport ly.img.android.pesdk.ui.panels.item.ToolItemimport ly.img.catalog.Rimport ly.img.catalog.examples.Exampleimport ly.img.catalog.resourceUri// <code-region>class PhotoAnnotation(private val activity: AppCompatActivity) : Example(activity) {override fun invoke() {// For this example only the sticker, text, and brush tool are enabled// highlight-menuval toolList = arrayListOf(ToolItem(StickerToolPanel.TOOL_ID,ly.img.android.pesdk.ui.sticker.R.string.pesdk_sticker_title_name,ImageSource.create(ly.img.android.pesdk.ui.R.drawable.imgly_icon_tool_sticker)),ToolItem(TextToolPanel.TOOL_ID,ly.img.android.pesdk.ui.text.R.string.pesdk_text_title_name,ImageSource.create(ly.img.android.pesdk.ui.R.drawable.imgly_icon_tool_text)),ToolItem(BrushToolPanel.TOOL_ID,ly.img.android.pesdk.ui.brush.R.string.pesdk_brush_title_name,ImageSource.create(ly.img.android.pesdk.ui.R.drawable.imgly_icon_tool_brush)))// highlight-menu// For this example only stickers suitable for annotations are enabled// highlight-stickersval annotationStickers = listOf(ImageStickerItem("imgly_sticker_shapes_arrow_02",ly.img.android.pesdk.assets.sticker.shapes.R.string.imgly_sticker_name_shapes_arrow_02,ImageSource.create(ly.img.android.pesdk.assets.sticker.shapes.R.drawable.imgly_sticker_shapes_arrow_02)),ImageStickerItem("imgly_sticker_shapes_arrow_03",ly.img.android.pesdk.assets.sticker.shapes.R.string.imgly_sticker_name_shapes_arrow_03,ImageSource.create(ly.img.android.pesdk.assets.sticker.shapes.R.drawable.imgly_sticker_shapes_arrow_03)),ImageStickerItem("imgly_sticker_shapes_badge_11",ly.img.android.pesdk.assets.sticker.shapes.R.string.imgly_sticker_name_shapes_badge_11,ImageSource.create(ly.img.android.pesdk.assets.sticker.shapes.R.drawable.imgly_sticker_shapes_badge_11)),ImageStickerItem("imgly_sticker_shapes_badge_12",ly.img.android.pesdk.assets.sticker.shapes.R.string.imgly_sticker_name_shapes_badge_12,ImageSource.create(ly.img.android.pesdk.assets.sticker.shapes.R.drawable.imgly_sticker_shapes_badge_12)),ImageStickerItem("imgly_sticker_shapes_badge_36",ly.img.android.pesdk.assets.sticker.shapes.R.string.imgly_sticker_name_shapes_badge_36,ImageSource.create(ly.img.android.pesdk.assets.sticker.shapes.R.drawable.imgly_sticker_shapes_badge_36)))// Create a custom sticker category for the annotation stickersval annotationStickersCategory = StickerCategoryItem("annotation_stickers","Annotation",ImageSource.create(ly.img.android.pesdk.assets.sticker.shapes.R.drawable.imgly_sticker_shapes_arrow_02),annotationStickers)// highlight-stickers// In this example, we do not need access to the Uri(s) after the editor is closed// so we pass false in the constructorval settingsList = PhotoEditorSettingsList(false).configure<LoadSettings> {// Set the source as the Uri of the image to be loadedit.source = activity.resourceUri(R.drawable.la)}// highlight-configsettingsList.configure<UiConfigMainMenu> {// Set custom tool listit.setToolList(toolList)}.configure<UiConfigSticker> {// Set custom sticker listit.setStickerLists(annotationStickersCategory)}// highlight-config// Start the photo editor using PhotoEditorBuilder// The result will be obtained in onActivityResult() corresponding to EDITOR_REQUEST_CODEPhotoEditorBuilder(activity).setSettingsList(settingsList).startActivityForResult(activity, EDITOR_REQUEST_CODE)// Release the SettingsList once donesettingsList.release()}override fun onActivityResult(requestCode: Int, resultCode: Int, intent: Intent?) {super.onActivityResult(requestCode, resultCode, intent)intent ?: returnif (requestCode == EDITOR_REQUEST_CODE) {val result = EditorSDKResult(intent)when (result.resultStatus) {EditorSDKResult.Status.CANCELED -> showMessage("Editor cancelled")EditorSDKResult.Status.EXPORT_DONE -> showMessage("Result saved at ${result.resultUri}")else -> {}}}}}// <code-region>
By leveraging the Android Annotation Editor SDK, you can empower your users to annotate photos with precision and ease, all within the familiar environment of your Android application. Start exploring the capabilities and customize the annotation experience to meet your app’s specific needs today!
500M+
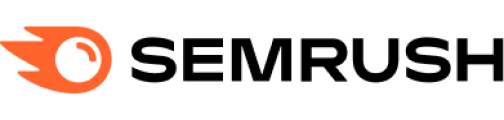
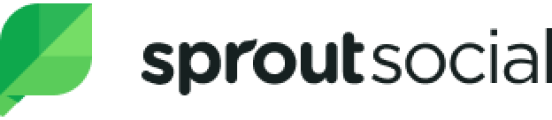
