PESDK/iOS/Guides
iOS Image Annotation SDK
The PhotoEditor SDK for iOS can be configured to allow evelopers to integrate advanced annotation features into iOS applications. While there is no dedicated annotation tool, the SDK can be easily configured to provide a powerful yet simple-to-use photo annotation tool. By enabling specific features, such as the brush, sticker, and text tools, you can create a customized annotation experience tailored to your needs.
Download Demo AppSystem Compatibility#
The Annotation Editor SDK for iOS requires iOS 11 or later. The SDK is optimized for modern iOS versions, ensuring compatibility and high performance across devices.
Key Capabilities of the iOS Annotation Editor SDK#
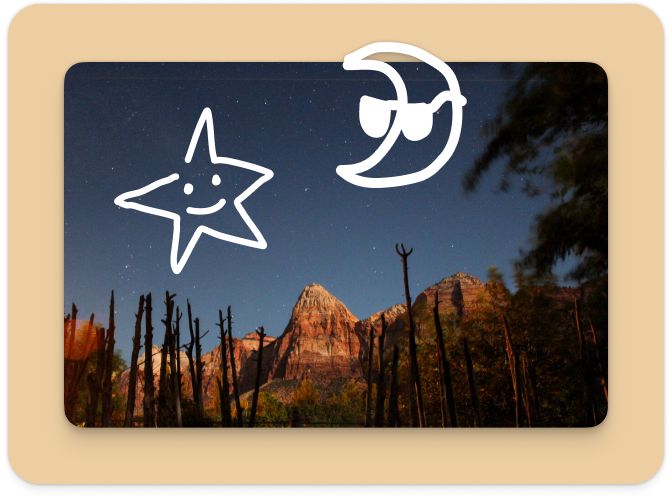
Brush Tool
Enable flexible marking and colored highlights on photos.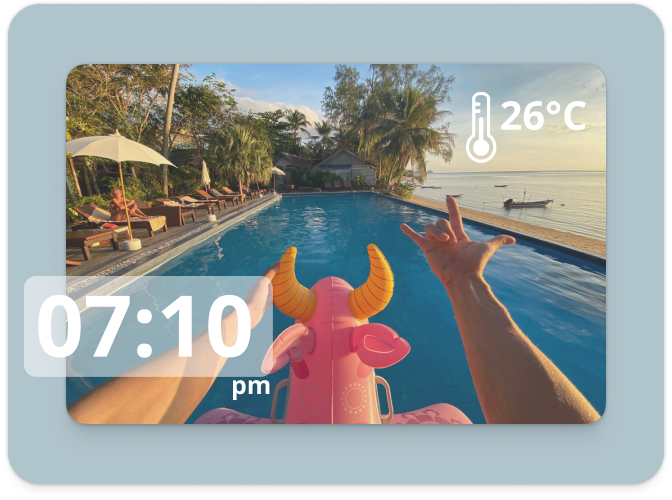
Sticker Tool
Add shapes such as arrows, tags, or badges for clear and effective annotations.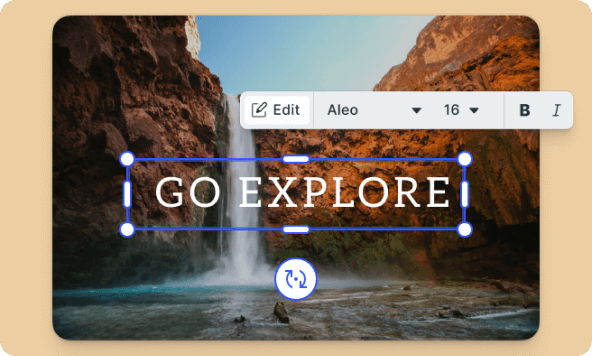
Text Tool
Provide additional information around annotation markers with custom text.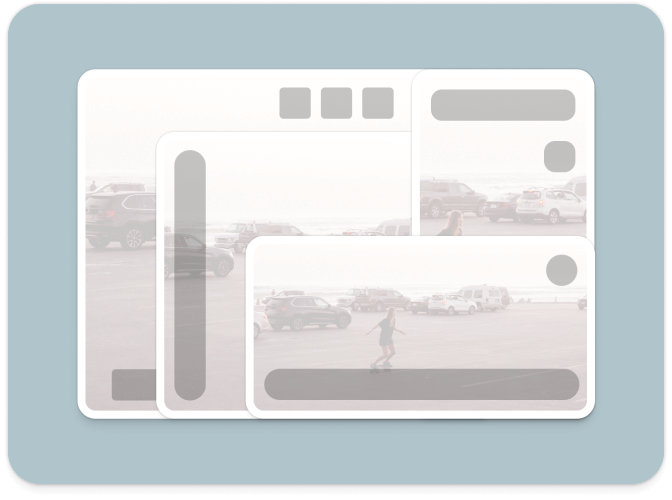
Customizable Sticker Sets
Configure the editor to include only annotation-specific stickers, ensuring a focused and streamlined user experience.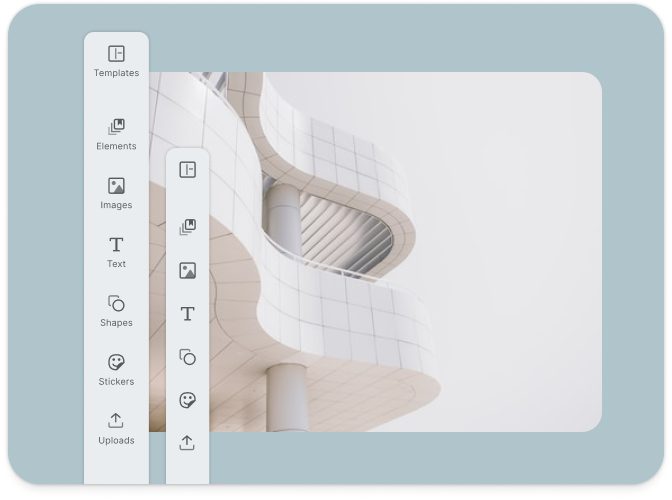
Configurable Menu Items
Tailor the editor’s menu to display only the tools necessary for annotation, simplifying the user interface.Essential Guides#
To help you get started and fully leverage the iOS Annotation Editor SDK, we've compiled a set of essential guides:
- How to Get Started: Integrate the editor into your project and configure it for annotation tasks.
- How to Configure the Menu Items: Learn how to include only the annotation-specific tools in the editor’s menu.
- How to Customize Stickers: Set up custom sticker categories with shapes such as arrows and badges for effective annotations.
- How to Use the Brush Tool: Implement the brush tool to allow users to highlight and mark areas of interest on photos.
- Understanding the SDK’s Architecture: Gain insights into the underlying architecture of the SDK to better understand its capabilities and how to utilize them.
- How to Handle Export and Errors: Manage the export process and handle errors gracefully within the annotation editor.
Customizing the iOS Annotation Editor SDK#
You can tailor the Annotation Editor SDK to fit your app’s specific annotation needs in several ways:
- Configuring the Tool Menu: Use the
ToolMenuItem
API to include only the brush, sticker, and text tools, creating a focused annotation experience. - Custom Sticker Categories: Load annotation-specific stickers, such as arrows and badges, from the app bundle, and group them meaningfully in the editor UI.
- Asset Catalog Configuration: Customize the asset catalog to include only the stickers relevant to annotations, ensuring that the editor remains simple and user-friendly.
Example Code#
Here's an example of how to configure the Annotation Editor SDK:
import PhotoEditorSDKimport UIKitclass PhotoAnnotationSolutionSwift: Example, PhotoEditViewControllerDelegate {override func invokeExample() {// Create a `Photo` from a URL to an image in the app bundle.let photo = Photo(url: Bundle.main.url(forResource: "LA", withExtension: "jpg")!)// For this example only the sticker, text, and brush tool are enabled.// highlight-menulet toolItems = [ToolMenuItem.createStickerToolItem(), ToolMenuItem.createTextToolItem(), ToolMenuItem.createBrushToolItem()]let menuItems: [PhotoEditMenuItem] = toolItems.compactMap { menuItem inif let menuItem = menuItem {return .tool(menuItem)}return nil}// highlight-menu// For this example only stickers suitable for annotations are enabled.// highlight-stickerslet stickerIdentifiers = ["imgly_sticker_shapes_arrow_02", "imgly_sticker_shapes_arrow_03", "imgly_sticker_shapes_badge_11", "imgly_sticker_shapes_badge_12", "imgly_sticker_shapes_badge_36"]let stickers = stickerIdentifiers.map { Sticker(imageURL: Bundle.imgly.resourceBundle.url(forResource: $0, withExtension: "png")!, thumbnailURL: nil, identifier: $0) }// Create a custom sticker category for the annotation stickers.let stickerCategory = StickerCategory(identifier: "annotation_stickers", title: "Annotation", imageURL: Bundle.imgly.resourceBundle.url(forResource: "imgly_sticker_shapes_arrow_02", withExtension: "png")!, stickers: stickers)// highlight-stickers// Create a `Configuration` object.// highlight-configlet configuration = Configuration { builder in// Add the annotation sticker category to the asset catalog.builder.assetCatalog.stickers = [stickerCategory]// Configure the `PhotoEditViewController`.builder.configurePhotoEditViewController { options in// Assign the custom selection of tools.options.menuItems = menuItems}}// highlight-config// Create and present the photo editor. Make this class the delegate of it to handle export and cancelation.let photoEditViewController = PhotoEditViewController(photoAsset: photo, configuration: configuration)photoEditViewController.delegate = selfphotoEditViewController.modalPresentationStyle = .fullScreenpresentingViewController?.present(photoEditViewController, animated: true, completion: nil)}// MARK: - PhotoEditViewControllerDelegatefunc photoEditViewControllerShouldStart(_ photoEditViewController: PhotoEditViewController, task: PhotoEditorTask) -> Bool {// Implementing this method is optional. You can perform additional validation and interrupt the process by returning `false`.true}func photoEditViewControllerDidFinish(_ photoEditViewController: PhotoEditViewController, result: PhotoEditorResult) {// The image has been exported successfully and is passed as an `Data` object in the `result.output.data`.// To create an `UIImage` from the output, use `UIImage(data:)`.// See other examples about how to save the resulting image.presentingViewController?.dismiss(animated: true, completion: nil)}func photoEditViewControllerDidFail(_ photoEditViewController: PhotoEditViewController, error: PhotoEditorError) {// There was an error generating the photo.print(error.localizedDescription)// Dismissing the editor.presentingViewController?.dismiss(animated: true, completion: nil)}func photoEditViewControllerDidCancel(_ photoEditViewController: PhotoEditViewController) {// The user tapped on the cancel button within the editor. Dismissing the editor.presentingViewController?.dismiss(animated: true, completion: nil)}}
By leveraging the iOS Annotation Editor SDK, you can empower your users to annotate photos with precision and ease, all within the familiar environment of your iOS application. Start exploring the capabilities and customize the annotation experience to meet your app’s specific needs today!
Ready to get started?
With a free trial and pricing that fits your needs, it's easy to find the best solution for your product.500M+
video and photo creations are powered by IMG.LY every month
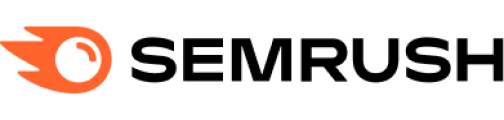
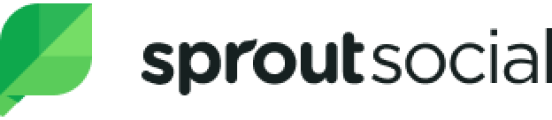
