Javascript Image Editor SDK
This CE.SDK configuration is fully customizable, offering a range of features that cater to various use cases, from simple photo adjustments, image compositions with background removal, to programmatic editing at scale. Whether you are building a photo editing application for social media, e-commerce, or any other platform, the CE.SDK Javascript Image Editor provides the tools you need to deliver a best-in-class user experience.
Explore Demo
Key Capabilities#
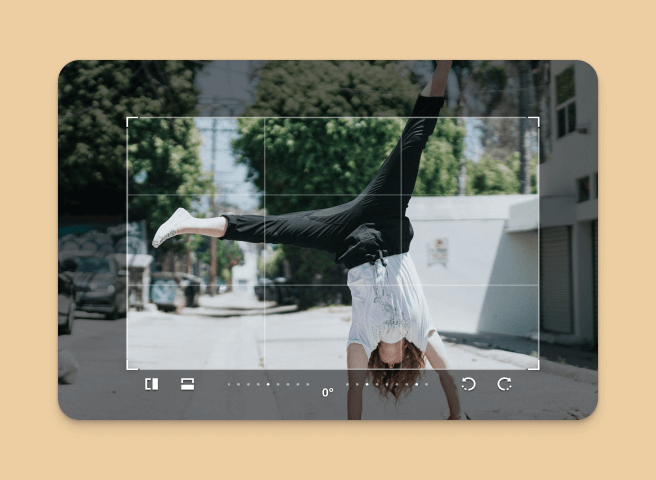
Transform
Easily perform operations like cropping, rotating, and resizing your design elements to achieve the perfect composition.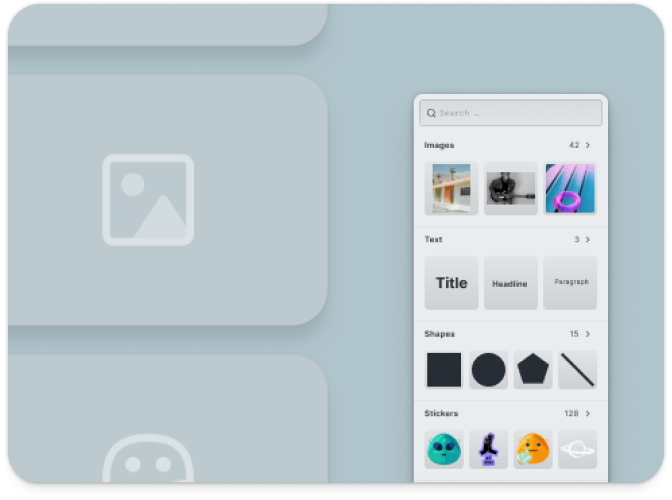
Asset Management
Import and manage stickers, images, shapes, and other assets to build intricate and visually appealing designs.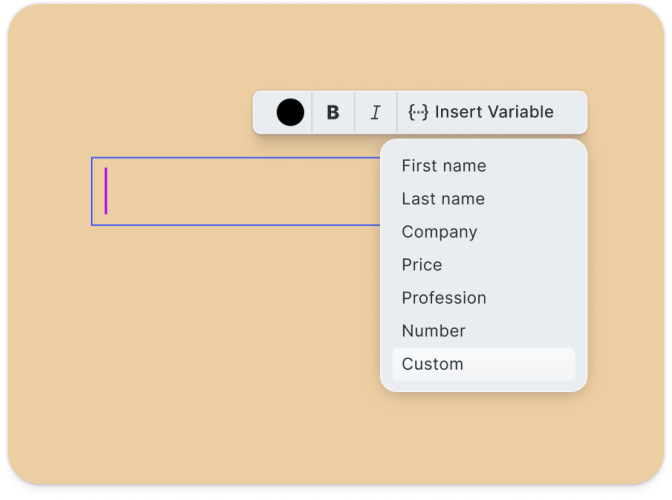
Text Editing
Add and style text blocks with a variety of fonts, colors, and effects, giving users the creative freedom to express themselves.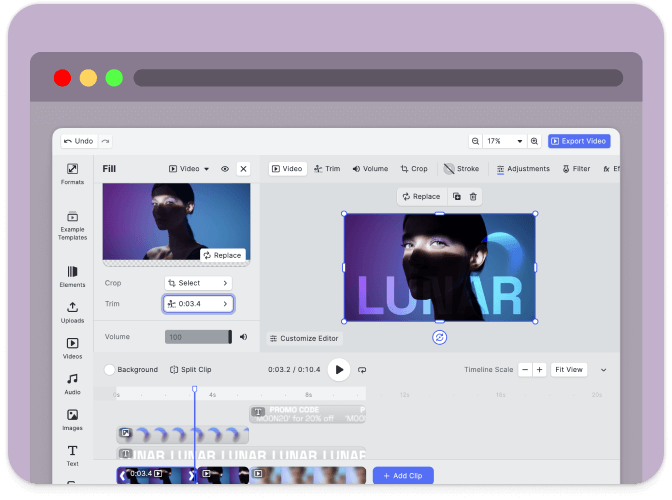
Client-Side Processing
All editing operations are performed directly in the browser, ensuring fast performance without the need for server dependencies.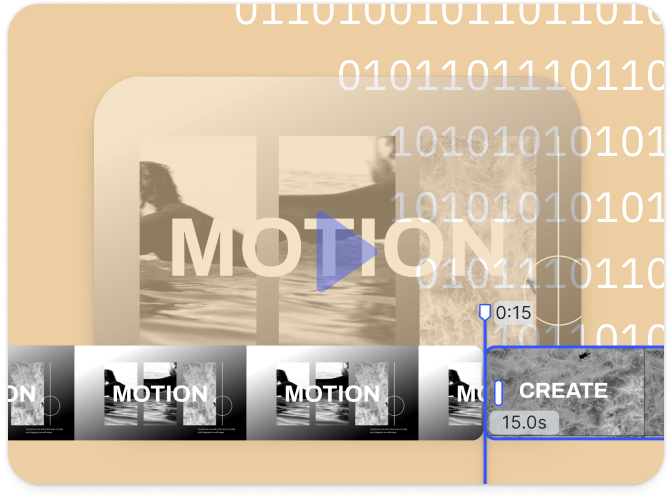
Headless & Automation
Programmatically edit designs using the engine API, allowing for automated workflows and advanced integrations within your application.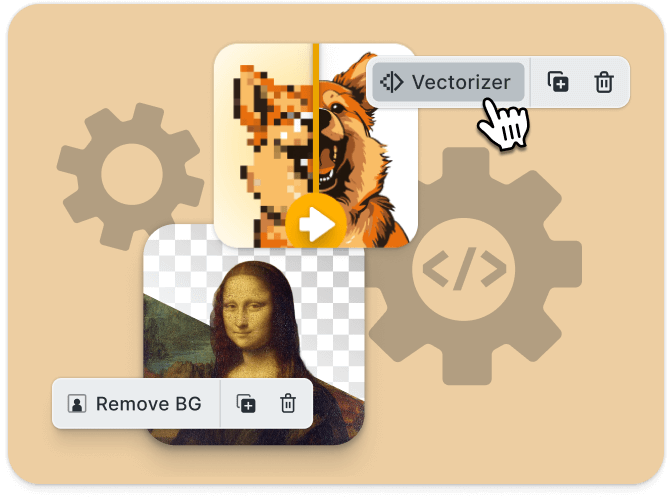
Extendible
Enhance functionality with plugins and custom scripts, making it easy to tailor the editor to specific needs and use cases.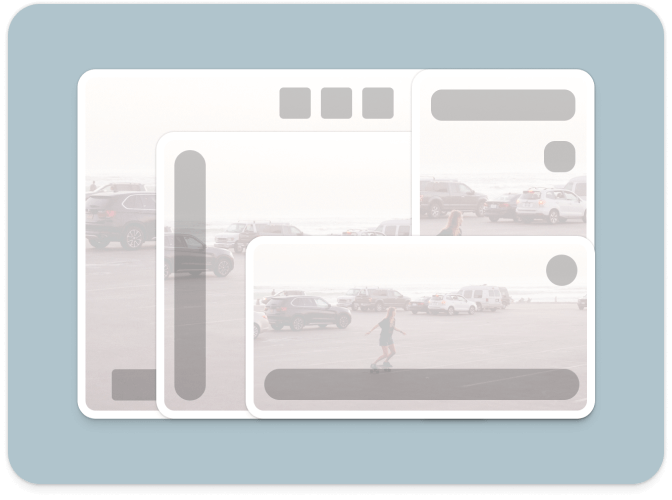
Customizable UI
Design and integrate custom user interfaces that align with your application’s branding and user experience requirements.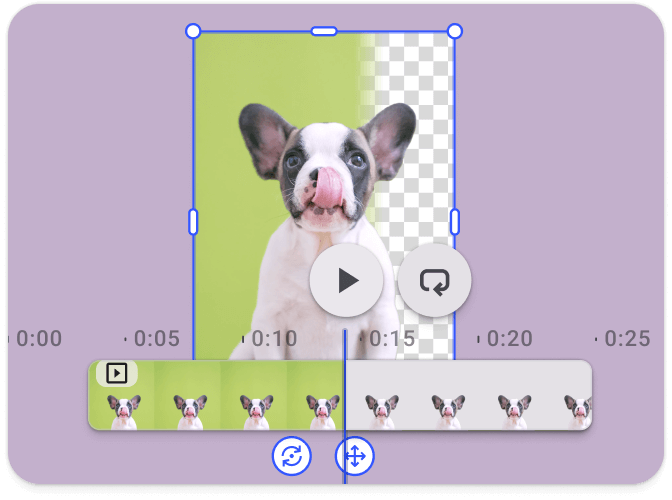
Background Removal
Utilize the powerful background removal plugin to allow users to effortlessly remove backgrounds from images, entirely on the Client-Side.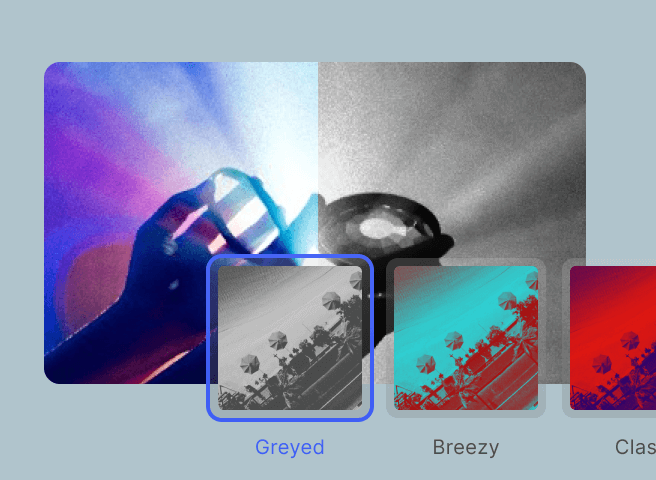
Filters & Effects
Choose from a wide range of filters and effects to add professional-grade finishing touches to photos, enhancing their visual appeal.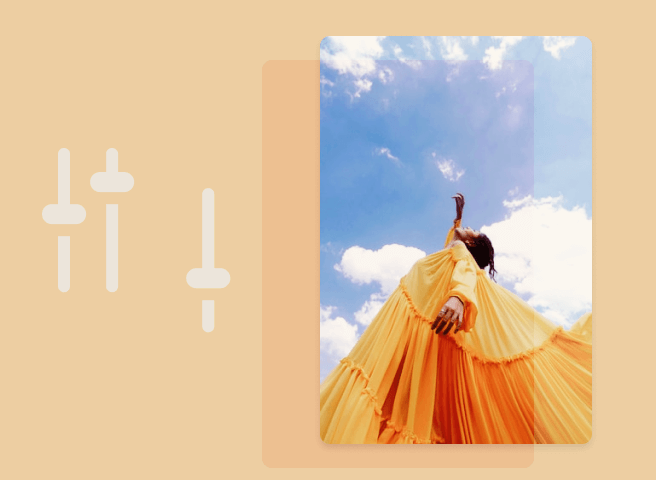
Size Presets
Access a variety of size presets tailored for different use cases, including social media formats and print-ready dimensions.Browser Support#
The CE.SDK Design Editor is optimized for use in modern web browsers, ensuring compatibility with the latest versions of Chrome, Firefox, Edge, and Safari. See the full list of supported browsers here.
Supported File Types#
IMG.LY's Creative Editor SDK enables you to load, edit, and save MP4 files directly in the browser without server dependencies.
The following file types can also be imported for use as assets during video editing:
MP3
MP4 (with MP3 audio)
M4A
AAC
JPG
PNG
WEBP
Individual scenes or assets from the video can be exported as JPG, PNG, Binary, or PDF files.
Getting Started#
If you're ready to start integrating CE.SDK into your Javascript application, check out the CE.SDK Getting Started guide. In order to configure the editor for an image editing use case consult our photo editor UI showcase and its reference implementation.
Understanding CE.SDK Architecture & API#
The following sections provide an overview of the key components of the CE.SDK photo editor UI and its API architecture.
If you're ready to start integrating CE.SDK into your application, check out our Getting Started guide or explore the Essential Guides.
CreativeEditor Photo UI#
The CE.SDK photo editor UI is a specific configuration of the CE.SDK which focuses the Editor UI to essential photo editing features. It further includes our powerful background removal plugin that runs entirely on the users device, saving on computing costs.
This configuration can be further modified to suit your needs, see the section on customization.
Designed for intuitive interaction, enabling users to create and edit designs with ease. Key components include:
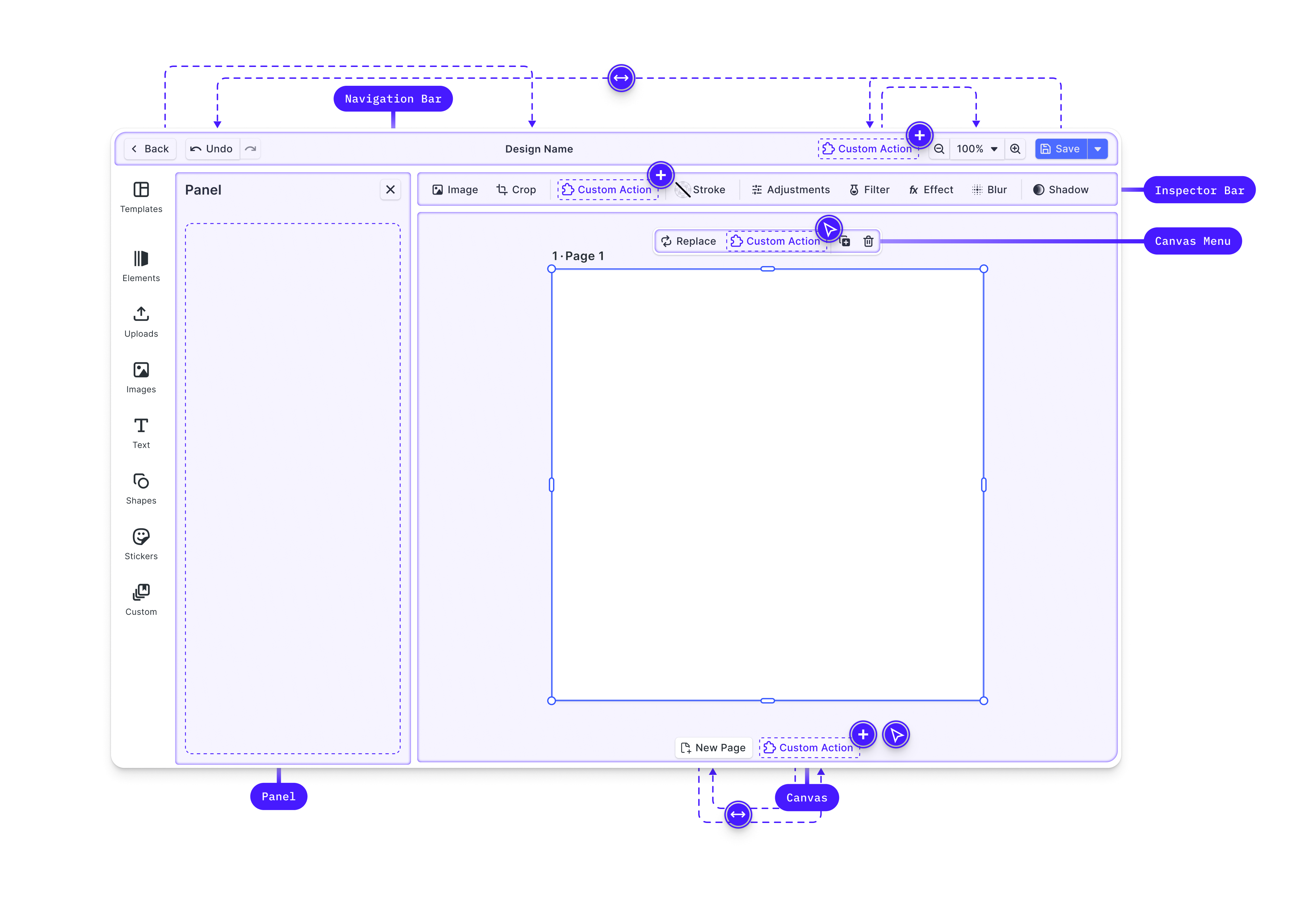
- Canvas: The primary workspace where users interact with their photo content.
- Dock: Provides access to tools and assets that are not directly related to the selected image or block, often used for adding or managing assets.
- Inspector Bar: Controls properties specific to the selected block, such as size, rotation, and other adjustments.
- Canvas Menu: Provides block-specific settings and actions such as deletion or duplication.
- Canvas Bar: For actions affecting the canvas or scene as a whole such as adding pages. This is an alternative place for actions such as zoom or redo/undo.
- Navigation Bar: Offers global actions such as undo/redo, zoom controls, and access to export options.
Learn more about interacting with and customizing the photo editor UI in our design editor UI guide.
CreativeEngine#
At the heart of CE.SDK is the CreativeEngine, which powers all rendering and design manipulation tasks. It can be used in headless mode or in combination with the CreativeEditor UI. Key features and APIs provided by CreativeEngine include:
- Scene Management: Create, load, save, and manipulate design scenes programmatically.
- Block Manipulation: Create and manage elements such as images, text, and shapes within the scene.
- Asset Management: Load and manage assets like images and SVGs from URLs or local sources.
- Variable Management: Define and manipulate variables for dynamic content within scenes.
- Event Handling: Subscribe to events such as block creation or selection changes for dynamic interaction.
API Overview#
CE.SDK’s APIs are organized into several categories, each addressing different aspects of scene and content management. The engine API is relevant if you want to programmatically manipulate images to create or modify them at scale.
Creating and Loading Scenes:
engine.scene.create();engine.scene.loadFromURL(url);Zoom Control:
engine.scene.setZoomLevel(1.0);engine.scene.zoomToBlock(blockId);
Creating Blocks:
const block = engine.block.create('shapes/rectangle');Setting Properties:
engine.block.setColor(blockId, 'fill/color', { r: 1, g: 0, b: 0, a: 1 });engine.block.setString(blockId, 'text/content', 'Hello World');Querying Properties:
const color = engine.block.getColor(blockId, 'fill/color');const text = engine.block.getString(blockId, 'text/content');
Managing Variables:
engine.variable.setString('myVariable', 'value');const value = engine.variable.getString('myVariable');
Managing Assets:
engine.asset.add('image', 'https://example.com/image.png');
Subscribing to Events:
engine.scene.onActiveChanged(() => {const newActiveScene = engine.scene.get();});
Basic Automation Example#
The following automation example shows how to turn an image block into a square format for a platform such as Instagram.
// Assuming you have an initialized engine and a selected block (which is an image block)// Example dimensionsconst newWidth = 1080; // Width in pixelsconst newHeight = 1080; // Height in pixels// Get the ID of the image block you want to resizeconst imageBlockId = engine.block.findByType('image')[0];// Resize the image blockengine.block.setWidth(imageBlockId, newWidth);engine.block.setHeight(imageBlockId, newHeight);// Optionally, you can ensure the content fills the new dimensionsengine.block.setContentFillMode(imageBlockId, 'Cover');
Customizing the CE.SDK Photo Editor#
CE.SDK provides extensive customization options, allowing you to tailor the UI and functionality to meet your specific needs. This can range from basic configuration settings to more advanced customizations involving callbacks and custom elements.
Basic Customizations#
Configuration Object: Customize the editor’s appearance and functionality by passing a configuration object during initialization.
const config = {baseURL: 'https://cdn.img.ly/packages/imgly/cesdk-engine/1.18.0/assets',license: 'your-license-key',locale: 'en',theme: 'light'};Localization: Adjust the editor’s language and labels to support different locales.
const config = {i18n: {en: {'libraries.ly.img.insert.text.label': 'Add Caption'}}};Custom Asset Sources: Serve custom sticker or shape assets from a remote URL.
UI Customization Options#
Theme: Choose between 'dark' or 'light' themes.
const config = {theme: 'dark'};UI Components: Enable or disable specific UI components as per your application’s needs.
const config = {ui: {elements: {toolbar: true,inspector: false}}};
Advanced Customizations#
For deeper customization, explore the range of APIs available for extending the functionality of the photo editor. You can customize the order of components, add new UI elements, and even develop your own plugins to introduce new features.
Plugins#
For cases where encapsulating functionality for reuse is necessary, plugins provide an effective solution. Use our guide on building plugins to get started, or explore existing plugins like Background Removal and Vectorizer.
Framework Support#
CreativeEditor SDK’s photo editor is compatible with any Javascript including, React, Angular, Vue.js, Svelte, Blazor, Next.js, Typescript. It is also compatible with desktop and server-side technologies such as electron, PHP, Laravel and Rails.
500M+
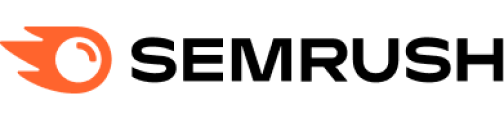
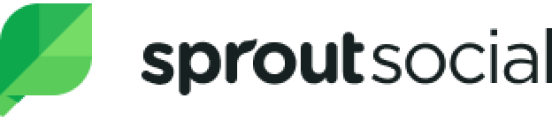
